Using custom visualizers for Actor components in UE4
If you've got an Actor component that needs some kind of in-editor representation, to make it easier to tweak settings like size or falloff, but you aren't deriving from something that would be rendered in editor like a StaticMeshComponent, you might want to use a custom component visualizer.
Let's say you have something like a ProceduralMeshComponent that draws a flat grid of polygons that will be deformed at runtime. You might want to have a representation of the grid like the following image:
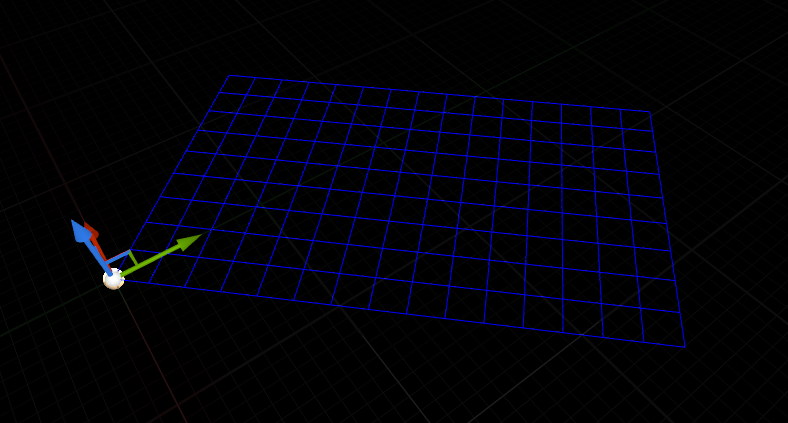
In order to do this we can create a FComponentVisualizer subclass.
There's a bunch of functions on this base class we can override but the only one we need to worry about for a simple visualization like this is the following:
class FGridVisualizer : public FComponentVisualizer
{
public:
/**
* Component = the component being drawn - cast this to your component to access its properties so you can draw your visualization
* View = the view to draw to
* PDI = the Primitive Draw Interface - we call our drawing functions on this
*/
void DrawVisualization(const UActorComponent* Component, const FSceneView* View,
FPrimitiveDrawInterface* PDI) override;
};
The implementation for our hypothetical grid visualizer might look something like this:
void FGridVisualizer::DrawVisualization(const UActorComponent* Component, const FSceneView* View, FPrimitiveDrawInterface* PDI)
{
//Cast the component reference to our class so we can read its properties
const UDeformableGridComponent* DrawComponent = Cast<UDeformableGridComponent>(Component);
//Iterate over all the nodes in our grid
for (UNode2D* Node: DrawComponent->Nodes)
{
//Draw a line between this node and its northern neighbour if it has one
if (Node->North)
{
PDI->DrawLine( FVector(Node->Position, 0), FVector(Node->North->Position,
0), FColor::Blue, SDPG_Foreground);
}
//Draw a line between this node and its eastern neighbour if it has one
if (Node->East)
{
PDI->DrawLine( FVector(Node->Position, 0), FVector(Node->East->Position,
0), FColor::Blue,SDPG_Foreground);
}
}
}
Given the visualization is a simple grid there's not really much to it. However, as with so many things we have in our editor module, we still need to register our component visualizer so that the engine knows to use it.
In your Editor module's StartupModule override, put the following code:
//Create a shareable instance of our visualizer - this will be used for all of our grid instances
TSharedPtr<FGridVisualizer> Visualizer = MakeShareable(new FGridVisualizer());
//Register that instance with the engine
GUnrealEd->RegisterComponentVisualizer(
UDeformableGridComponent::StaticClass()->GetFName(), Visualizer);
We need to tell the engine that our editor module is going to use component visualizers, so go ahead and add a dependency in your Build.cs file:
PrivateDependencyModuleNames.AddRange(
new string[]
{
"UnrealEd",
//other dependencies here
"ComponentVisualizers"
}
);
In addition to this, we need to get our editor module loaded earlier in the engine initialization process. Add the following to the uproject file:
{
"Name": "MyGameEditor",
"Type": "Editor",
"LoadingPhase": "PostEngineInit"
}
Rebuild and relaunch the editor and when you select an Actor that contains your custom component, you should see your new visualization!